Flutter Flame Game development
Develop mobile games with one codebase by using Flutter and the flame engine.
Posted by

Related reading
The best way to play Block blast!
Developing mobile games is challenging. But there is
Flutter is a popular cross-platform framework. It's a great tool to develop Android and iOS games from one codebase - and ShipGameNow builds on it!
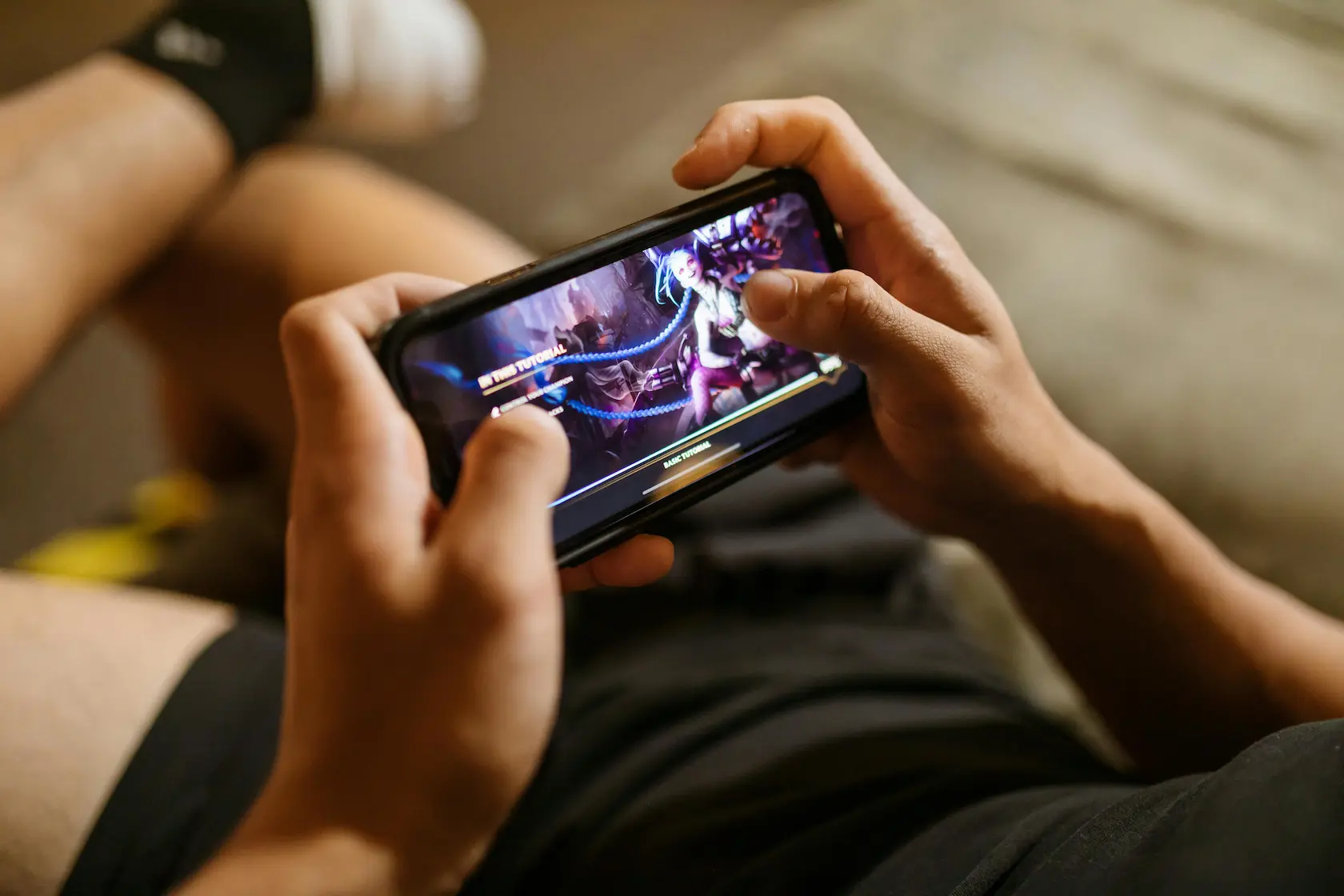
Leveling Up: The Mobile Game Challenges Every Indie Developer Faces
As an indie game developer, venturing into the world of mobile games can feel a lot like tackling a roguelike dungeon. Every turn brings new surprises, and every boss (or bug) seems more daunting than the last. Let’s break down the common challenges indie devs face in this high-stakes adventure—and maybe drop a few power-ups along the way.
How to Create a Simple Mobile Game with Flutter and Flame
Creating a mobile game can be a fun and rewarding experience, especially with tools like Flutter and the Flame engine. Flutter provides a powerful cross-platform framework, while Flame extends it with game-specific features.
1. Setting Up Your Development Environment
Ensure you have Flutter installed. If you haven't set it up yet, follow the official Flutter installation guide.
flutter create flame_game cd flame_game flutter pub add flame flutter pub add flame_audio
2. Creating the Game Structure
import 'package:flame/game.dart';
import 'package:flutter/widgets.dart';
class SimpleGame extends FlameGame {
@override
Future<void> onLoad() async {
super.onLoad();
// Load assets and initialize game objects here
}
}
void main() {
runApp(GameWidget(game: SimpleGame()));
}
3. Adding a Player Character
Load the Player Sprite
flutter: assets: - assets/images/player.png
Create the Player Component
import 'package:flame/components.dart';
import 'package:flame/flame.dart';
import 'package:flame/game.dart';
class Player extends SpriteComponent {
@override
Future<void> onLoad() async {
sprite = await Sprite.load('player.png');
size = Vector2.all(50.0);
position = Vector2(100, 100);
}
}
4. Handling User Input
import 'package:flame/input.dart';
import 'package:flutter/services.dart';
class SimpleGame extends FlameGame with KeyboardEvents {
@override
void onKeyEvent(RawKeyEvent event, Set<LogicalKeyboardKey> keysPressed) {
if (event is RawKeyDownEvent) {
if (event.logicalKey == LogicalKeyboardKey.arrowLeft) {
player.x -= 10;
} else if (event.logicalKey == LogicalKeyboardKey.arrowRight) {
player.x += 10;
}
}
}
}
5. Adding Collisions and Physics
flutter pub add flame_forge2d
Create a physics-based player component and a platform.
6. Adding Sounds and Effects
import 'package:flame_audio/flame_audio.dart';
void playJumpSound() {
FlameAudio.play('jump.wav');
}
7. Polishing and Deployment
- Testing & Debugging (3-5 hours)
- UI/UX Improvements (3-6 hours)
- Publishing to the Play Store/App Store (4-8 hours)
Total Estimated Development Time
Task | Estimated Time |
---|---|
Setting up Flutter & Flame | 30 minutes |
Creating the game structure | 1 hour |
Adding player character | 2 hours |
Handling user input | 2 hours |
Adding physics and collisions | 3-5 hours |
Adding sound effects | 1-2 hours |
Polishing & Deployment | 10-15 hours |
Total Time | 20-30 hours |
Conclusion
With Flutter and Flame, you can create simple 2D games quickly. A basic game can be developed in a weekend or a few weeks part-time. Want to take it further? Try adding animations, leaderboards, or multiplayer features!
Happy coding! 🚀
Ship your mobile game in 5 minutes!